'It is just the concept of having a memory fading over time. Light a light gradually getting duller and duller...'
I decided to then model this using arduino: whereby pressing a button lights up an LED which gradually fades.
Requirements:
A button, a light, some code.
Software development technique: RAPID (Rapid Application Development facilitates fast prototyping and minimal planning which makes it easy to change requirements if desired). This allows for easy project expansion.
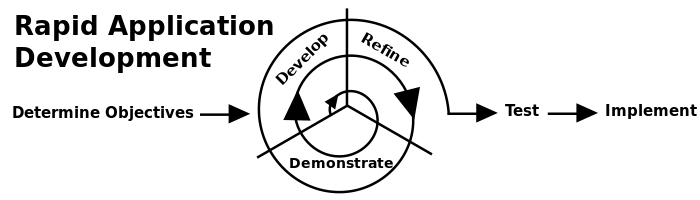
I have decided on the requirements, and will work on it in a modular fashion and then put the pieces together.
Step 1: Wiring up a button and LED
Step 2: Getting a light to turn on when the button is pushed
Step 3: Lighting slowly fading off,
Step 4: Getting it to light and re-light whenever the button is pushed.
Steps 1 and 2 were simple. I looked up pulse width modulation to get the LED to slowly turn off. I incorporated some sample fading code from http://arduino.cc/en/Tutorial/Fade and tweaked it to just turn off. An initial error was using a normal pin rather than a PWM pin which meant this it didnt slowly fade on and off; only turned on and off completely. This was noticed and rectified. The sample code faded on and off, but i wanted it to just fade off so i removed the looping code.
// reverse the direction of the fading at the ends of the fade:
if (brightness == 0 || brightness == 255) {
fadeAmount = -fadeAmount ;
}
I then had to incorporate counter code which would exit the loop after one fade.
int counter = 0; was added at the top
added an 'if' comparison around the existing code which will allow me increase the counter by one at the end of the loop and therefore make the if statement false until reset. This will mean it only fades once.
if (counter < 52) {
analogWrite(ledPin, brightness);
// change the brightness for next time through the loop:
brightness = brightness - fadeAmount;
// wait for 30 milliseconds to see the dimming effect
delay(30);
counter++;
}
I then had to incorporate a trigger button into this code. I added a button input and wired it up. I tested it with some basic serial.write() code.
Note: I comment my own code to make it easier for me to understand. This is shown as '//' in the arduino language.
The next step was getting it to fade whenever the button was pushed. Now, the problem is that i'll need state change detection which was easily to find an example of, but with a momentary switch like the one that I have, it quickly turns off. So, the fade code would only have a split second to run and wouldn't work as desired.
To combat this i'd have to get the button to manipulate another variable, which is named 'counterNew'. I originally tested this using blink code rather than fade code as it was simpler. It was successful and i could easily manipulate it blink on and off as many times as i liked.
Underneath the state change detection code i added ' counterNew = 1;'. This will set the variable to 1 whenever the button is pressed fully (up and down). If i did not use this code, i would have to hold the button down to notice the fading. I then added this code at the bottom of the page outside of the state change loop.
if (counterNew < 52) { // fades by 52 to turn light off completely
digitalWrite(ledPin, HIGH);
delay(50);
digitalWrite(ledPin, LOW);
delay(50); // will be reset as a global variable
counterNew ++;
}
This blinks the led on and off 51 times.
The next step is to add fading code in its place. This was a little difficult, but it was eventually incoporated.
The final system turns a light off slowly. It could be incorporated into the paired distance sensing system but with multiple inputs. It could also be used by a single person i.e. a night light which slowly turns itself off. There does not necessarily have to be 'presence indicating' element to the system and it can take many forms. I feel that this prototype could be expanded upon it itself and sums up transient memory in itself.
This is a photograph of the prototype:
Expanding it to xBee systems.....
This is very similar to previous xBee models that i've made. All i need to do is send a letter to serial when the button is pushed on one xBee Arduino, which will then execute on the other xBee arduino.
Arduino A code:
if (buttonState != lastButtonState) {
Serial.print('k');
and remove the LED executing code on this arduino
Arduino B code;
if(Serial.read() = 'k');
//then
counterNew = 1;
//execute rest of code
Modelling this in the real world....
In the tutorial last week the concept of this been less functional and more just for fun was proposed... Also was the idea of the idea of false memories. How could this be used in this system?
Source code.
int brightness; // how bright the LED is
int fadeAmount = 5; // how many points to fade the LED by
const int ledPin = 9;
int counter = 0;
const int buttonPin = 6; // the number of the pushbutton pin
int buttonPushCounter = 0; // counter for the number of button presses
int buttonState = 0; // current state of the button
int lastButtonState = 0; // previous state of the button
int counterNew;
int delayamount = 20; //the fade speed
void setup() {
pinMode(ledPin, OUTPUT); //led
pinMode(buttonPin, INPUT); //pushbutton
Serial.begin(9600);
}
void loop() {
buttonState = digitalRead(buttonPin);
// counter loop which fades
if (buttonState != lastButtonState) {
// if the state has changed, increment the counter
counterNew = 1;
brightness = 255; // how bright the LED is
if (buttonState == LOW) {
// if the current state is HIGH then the button
// wend from off to on:
buttonPushCounter++;
Serial.println("on");
Serial.print("number of button pushes: ");
Serial.println(buttonPushCounter, DEC);
}
else {
// if the current state is LOW then the button
// wend from on to off:
Serial.println("off");
}
}
// save the current state as the last state,
//for next time through the loop
lastButtonState = buttonState;
//modulus
if (buttonPushCounter % 4 == 0) {
// digitalWrite(ledPin, HIGH);
} else {
// digitalWrite(ledPin, LOW);
}
if (counterNew < 53) {
analogWrite(ledPin, brightness);
// change the brightness for next time through the loop:
brightness = brightness - fadeAmount;
// wait for 30 milliseconds to see the dimming effect
delay(delayamount);
counterNew ++;
}
/*
if (counter < 52) {
analogWrite(ledPin, brightness);
// change the brightness for next time through the loop:
brightness = brightness - fadeAmount;
// wait for 30 milliseconds to see the dimming effect
delay(30);
counter++;
}
*/
Serial.print(counter);
}
No comments:
Post a Comment